Programming errors can be frustrating, especially when they disrupt the flow of development. One such error, “error call to a member function getCollectionParentId() on null,” often perplexes developers due to its technical nature. In this article, we will break it down, explore the causes, and provide actionable solutions to address it effectively.
What Does This Error Mean?
The error “error call to a member function getCollectionParentId() on null” occurs when your code tries to invoke the getCollectionParentId()
function on an object that is null
. In simpler terms, you’re attempting to access a method or property of an object that doesn’t exist or has not been properly instantiated.
Key Reasons Behind the Error
Understanding why this error happens is essential for resolving it. Here are the primary causes:
1. Null Object Reference
- The object you are trying to use hasn’t been assigned a valid value.
- It might be null due to incomplete initialization or errors in prior operations.
2. Incorrect Object Type
- The object may not be of the expected type.
- If the
getCollectionParentId()
method is not defined in the object’s class, calling it will result in an error.
3. Logical Gaps in Code
- Logic flaws, such as missing checks for null values before calling methods, often lead to such errors.
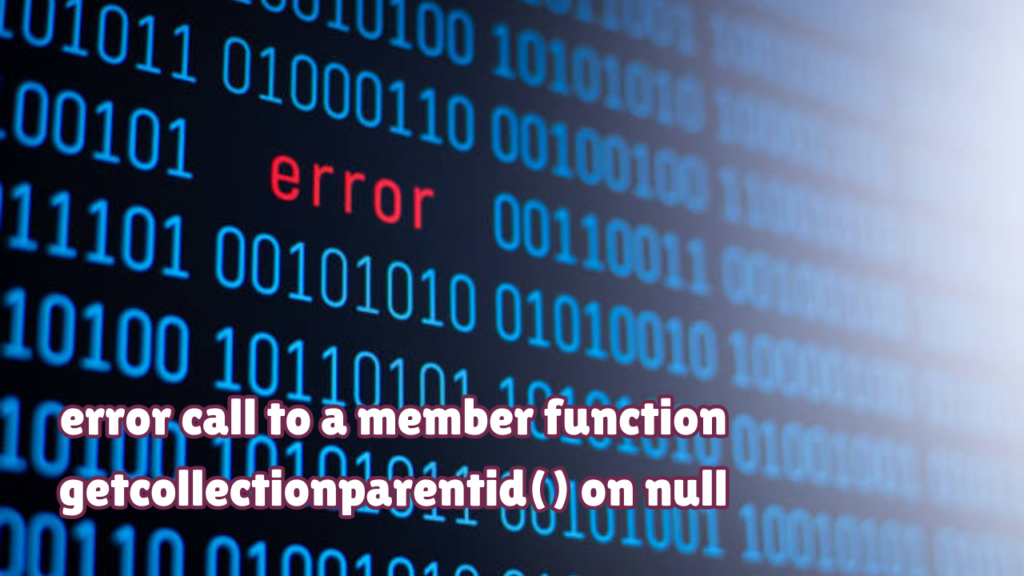
Steps to Debug and Fix the Error
1. Verify Object Initialization
Before invoking the getCollectionParentId()
function, ensure that the object is initialized:
phpCopy codeif ($object !== null) {
$parentId = $object->getCollectionParentId();
} else {
echo "The object is null.";
}
This conditional check prevents the function call if the object is null.
2. Inspect the Object Type
- Use debugging tools or
var_dump()
in PHP to inspect the object:phpCopy codevar_dump($object);
- Confirm that the object is of the correct type and contains the
getCollectionParentId()
method.
3. Review the Code Logic
Examine the code structure and flow to identify:
- Where the object is being assigned.
- Potential points where it could be set to null unintentionally.
4. Debug with a Debugger
A step-by-step debugging session can reveal:
- Where the object becomes null.
- Logical errors causing the issue.
5. Use Default or Fallback Values
If there’s a possibility that the object might be null, use a fallback mechanism:
phpCopy code$parentId = $object !== null ? $object->getCollectionParentId() : 'default_value';
Example Cod
Here’s a practical example to illustrate the resolution process:
phpCopy code// Example: Validating object before accessing the method
if ($collection !== null) {
$parentId = $collection->getCollectionParentId();
echo "Parent ID: " . $parentId;
} else {
echo "Error: Collection object is null.";
}
This ensures the code remains robust and handles unexpected null values gracefully.
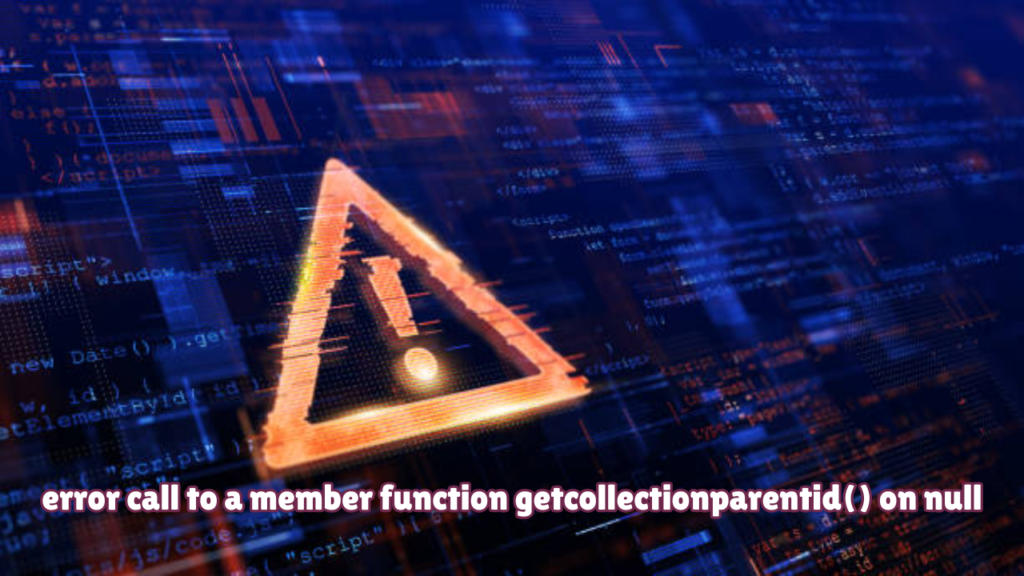
Best Practices to Prevent This Error
- Always Initialize Objects:
- Assign default values where possible.
- Ensure object creation before invoking methods.
- Validate Input:
- Check all inputs that could impact object initialization.
- Use Exception Handling:
- Catch errors gracefully and log useful information.
try { $parentId = $object->getCollectionParentId(); } catch (Exception $e) { echo "Error occurred: " . $e->getMessage(); }
- Follow Object-Oriented Design Principles:
- Properly structure your classes and objects to avoid type mismatches.
Why Debugging Matters
Errors like “error call to a member function getCollectionParentId() on null” can appear intimidating, but they often point to fundamental issues in code structure. By addressing these systematically, you not only resolve the immediate problem but also improve the overall quality and reliability of your code.
Also Read : healthtdy.xyz: Your Gateway to Better Living
Conclusion
The “error call to a member function getCollectionParentId() on null” is a common yet solvable issue. With careful debugging, validation, and adherence to best practices, you can prevent and handle this error efficiently. Remember, robust code is the result of proactive error handling and clear logic flow.
By applying the tips and techniques shared in this article, you can confidently tackle this error and create code that is both functional and maintainable.